Content Management Systems (CMS) are widely used across various industries to
create, edit, and manage digital content. These platforms have evolved with
time, offering more advanced features, functionality, and integration options.
One of the most recent and promising developments in this area is the
integration of ChatGPT, a powerful AI language model, into existing CMS
systems.
Imagine a world where content management is no longer a tedious,
time-consuming
process. A world where your CMS system can understand context, translate
languages, and even generate content on its own. Sounds like a dream come
true,
right? Well, thanks to ChatGPT, this dream is quickly becoming a reality. In
this article, we’ll explore how ChatGPT, the AI-powered language model, can
revolutionize content management systems by swooping in like a superhero to
save
the day — and your content!
ChatGPT: A Brief Overview
ChatGPT, developed by OpenAI, is a cutting-edge AI language model that can
generate human-like text based on the input provided. It leverages advanced
machine learning algorithms and natural language processing capabilities to
understand context, generate responses, and even translate languages. This
technology has immense potential for integration into various digital platforms,
including CMS systems.
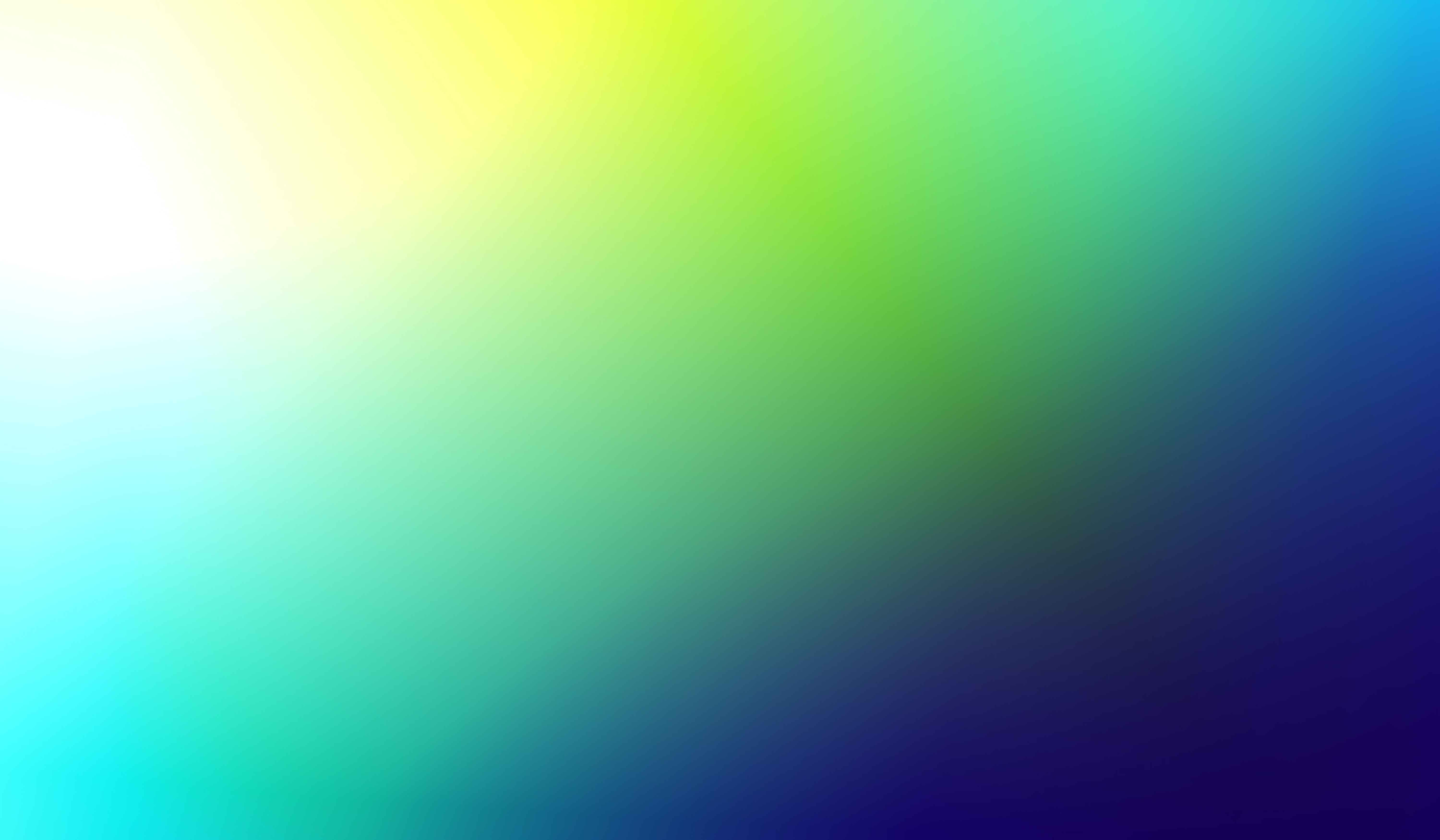
How ChatGPT Can Enhance CMS Systems
Picture ChatGPT as the superhero sidekick to your CMS. It’s here to help you
tackle content management challenges and make your life easier. Let’s take a
look at some of the extraordinary abilities it brings to the table:
1. Autofilling Fields
ChatGPT can be used to autofill fields within a CMS, saving time and effort
for content creators and editors. By analysing the context of a specific
entry, the AI can generate relevant content, titles, descriptions, tags, or
other metadata required by the system.
2. Completing Missing Data
In some cases, CMS users might have incomplete data sets or face difficulty
in finding the right information to fill specific fields. ChatGPT can analyze
the available data and generate the missing information, ensuring a more
comprehensive and accurate content record.
3. Translations
With its advanced language processing capabilities, ChatGPT can provide
translations for content within a CMS, allowing content creators to easily
publish their work in multiple languages. This feature can help businesses
reach a wider audience and improve global engagement.
4. Content Generation
ChatGPT’s ability to generate human-like text can be used to create new
content within a CMS. For example, it can generate blog posts, product
descriptions, or other content types based on the user’s input, substantially
reducing the time and effort required.
5. Content Optimisation
ChatGPT can analyse existing content and suggest improvements to enhance
readability, SEO, and user engagement. It can help identify and fix grammar,
spelling, and punctuation issues, as well as optimize keyword usage,
headings, and metadata to improve search engine rankings.
6. Content Personalisation
By leveraging user data and analytics, ChatGPT can generate personalized
content recommendations for individual users. This could include tailoring
article suggestions, product recommendations, or other content based on user
preferences, browsing history, and demographics, leading to a more engaging
user experience.
7. Content Summarization
ChatGPT can automatically generate concise summaries of articles, reports, or
other long-form content within the CMS. This feature can help users quickly
understand the main points of a piece of content without having to read the
entire text, making it easier to browse and navigate the website.
8. Automated Content Curation
ChatGPT can be used to automatically curate content for newsletters,
roundups, or other compilation-style posts. By analyzing user preferences,
topic relevance, and content popularity, ChatGPT can generate a list of
suitable articles or pieces of content to be included in such compilations,
saving time and effort for content creators and editors.
9. Comment Moderation
Integrating ChatGPT with a CMS can help facilitate automated comment
moderation. The AI can analyze user-generated comments for spam,
inappropriate language, or other violations of community guidelines, and
either flag or remove them automatically, ensuring a safe and welcoming
environment for users.
10. FAQ Generation
ChatGPT can assist in creating Frequently Asked Questions (FAQs) sections
for websites by analyzing existing content and user queries. This feature
can help businesses quickly address common concerns and questions from their
audience and improve overall user experience.
11. Topic Research
ChatGPT can be used to generate content ideas and topics for articles, blog
posts, or other forms of content. By analyzing trends, user interests, and
related content, the AI can suggest fresh and engaging topics for content
creators to explore.
These are just a few examples of the many potential use cases for integrating
ChatGPT with a CMS. As AI technology continues to evolve, the possibilities for
enhancing content management and user experiences will only grow.
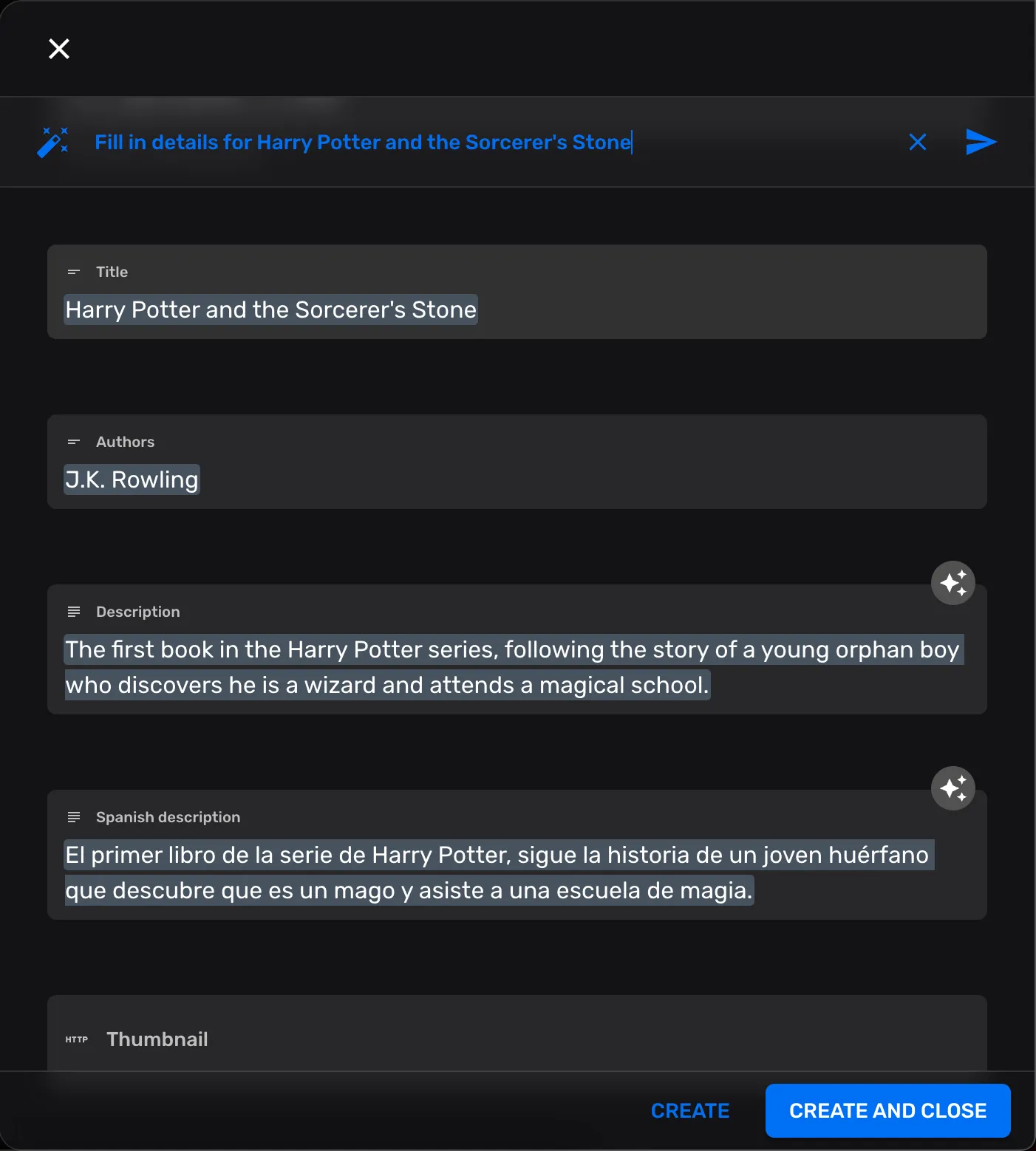
As impressive as ChatGPT’s capabilities are, it’s essential to ensure that its
outputs can be effectively integrated into a CMS platform. FireCMS, an
open-source CMS, demonstrates a successful approach to extracting structured
data from ChatGPT outputs and adapting it to any given data entity structures.
FireCMS can be configured to communicate with ChatGPT, sending input queries and
receiving generated content. By implementing custom parsers and data processing
tools, FireCMS can extract the relevant information from ChatGPT’s outputs and
adapt it to the required data structure. This process allows FireCMS to
seamlessly integrate ChatGPT-generated content into its existing content
management workflow.
The integration of FireCMS with OpenAI is completely free to try in your own
project, no subscriptions required!
https://youtube.com/shorts/WIfHyvDRam0
Conclusion
The integration of ChatGPT into CMS systems has the potential to revolutionise
content management, making it more efficient, accurate, and versatile. By
autofilling fields, completing missing data, providing translations, and
generating content, ChatGPT can streamline content creation and management
processes while offering a higher degree of automation.
FireCMS showcases a successful approach to integrating ChatGPT into a CMS
platform, setting the stage for other CMS systems to follow suit. As AI
technology continues to advance, the potential for further improvements and
innovations in content management is limitless.